There are many factors to consider when choosing an architecture for an iOS app, including the size and complexity of the app, the type of data being managed, the target audience, and the goals of the project. Some common architectures that are used in iOS app development include:
- MVC (Model-View-Controller): This is a classic architecture that has been widely used in iOS development. It involves separating the app into three main components: the model, which represents the data and business logic; the view, which represents the user interface; and the controller, which mediates communication between the model and the view.
- MVVM (Model-View-ViewModel): This architecture is similar to MVC, but it introduces an additional layer called the ViewModel, which is responsible for preparing and formatting data for the view to display. This can help to reduce the amount of code in the view and make it easier to test and maintain.
- VIPER (View, Interactor, Presenter, Entity, and Router): This architecture is designed to be scalable and modular, with each component serving a specific purpose. The view represents the user interface, the interactor manages business logic, the presenter prepares data for the view, the entity represents the data model, and the router handles navigation.
Why do a lot of developers prefer VIPER?
There are several reasons why some iOS developers may prefer to use the VIPER architecture when building apps:
Separation of concerns: VIPER promotes separation of concerns by clearly defining the responsibilities of each component and separating the user interface, business logic, and data model into distinct layers. This can make it easier to understand the codebase and make changes to specific parts of the app without affecting other parts.
Improved testability: Each component in a VIPER architecture can be tested in isolation, which can make it easier to write unit tests and ensure that the app is working as intended.
Modularity: The VIPER architecture is designed to be modular, which means that different parts of the app can be developed and tested independently and then integrated together. This can make it easier to scale the app and add new features over time.
Improved maintainability: The clear separation of responsibilities in a VIPER architecture can make it easier to maintain the codebase over time, as it is easier to understand how different parts of the app fit together.
So VIPER is a scalable and modular approach to building iOS apps that helps to separate responsibilities and improve the testability and maintainability of the codebase.
A brief overview of how the VIPER architecture works
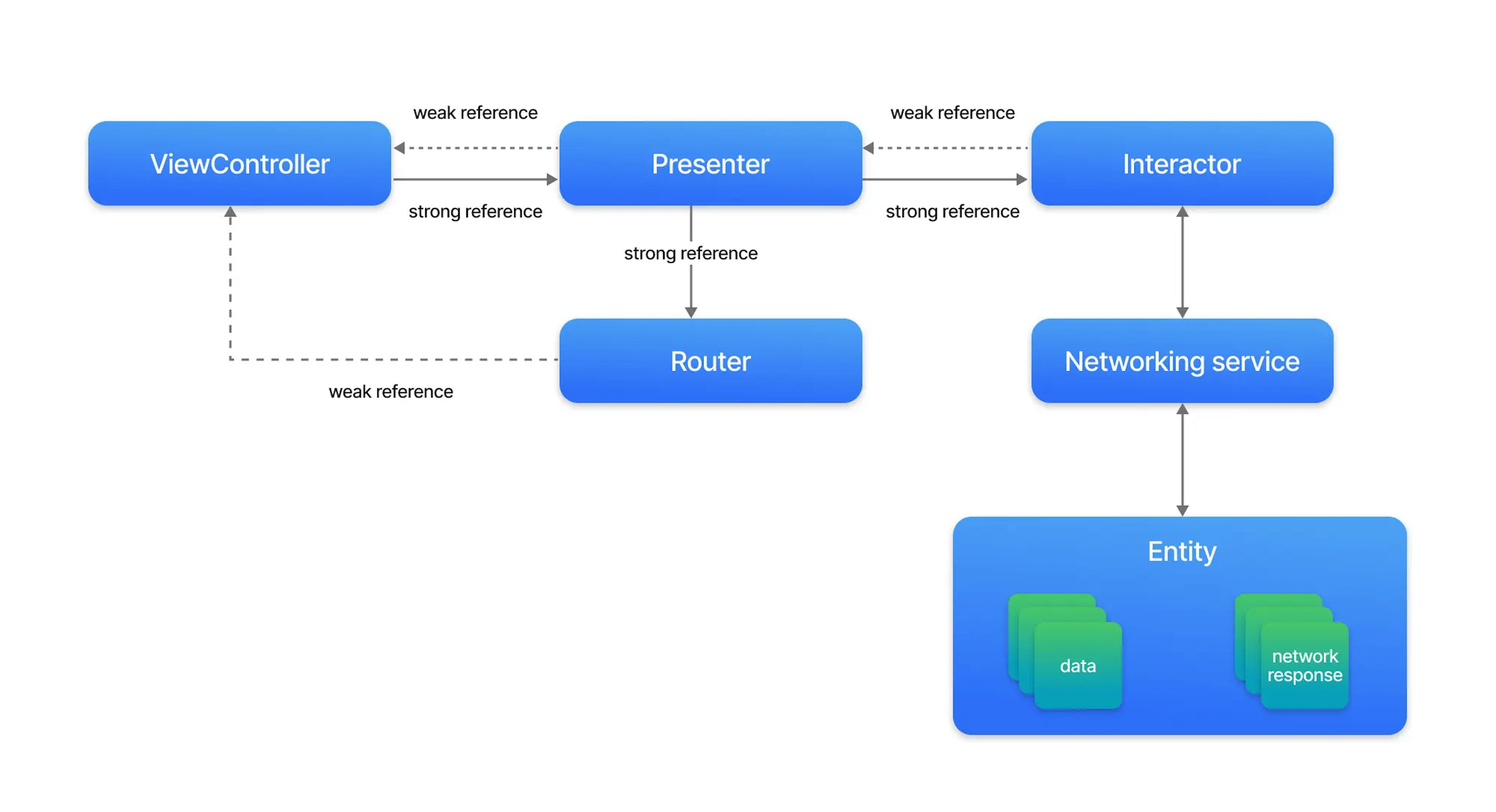
Structure of VIPER Module
Here is a brief overview of how the VIPER architecture works:
- View: The view is the user interface of the app, responsible for displaying data and handling user input.
- Interactor: The interactor manages the business logic of the app and communicates with the presenter to retrieve and format data for the view.
- Presenter: The presenter prepares data for the view to display and handles interactions from the view, updating the model as necessary.
- Entity: The entity represents the data model of the app and is responsible for storing and manipulating data.
- Router: The router handles navigation between different screens and modules of the app.
To implement VIPER in an iOS app, you will need to create separate classes for each of these components and define their responsibilities. You can then connect these classes using protocols to facilitate communication and data flow.
Here is an example of how you might set up a VIPER module in an iOS app:
In this example, each of the VIPER components is defined as a protocol with a corresponding class implementation. The view, interactor, and router are connected to the presenter using weak properties, and the presenter is connected to the view and interactor using strong properties.
You can then use the presenter to mediate communication between the view and the interactor, passing data and triggering actions as needed. The router is responsible for handling navigation between different screens and modules in the app.
To summing up
VIPER is designed to help developers create scalable and modular apps that are easier to test, maintain, and extend over time.
In the VIPER architecture, the view represents the user interface of the app and is responsible for displaying data and handling user input. The interactor manages the business logic of the app and communicates with the presenter to retrieve and format data for the view. The presenter prepares data for the view to display and handles interactions from the view, updating the model as necessary. The entity represents the data model of the app and is responsible for storing and manipulating data. The router handles navigation between different screens and modules of the app.
One of the main benefits of using the VIPER architecture is that it helps to clearly define the responsibilities of each component and promotes separation of concerns. This can make it easier to understand the codebase and make changes to specific parts of the app without affecting other parts. It can also help to improve the testability of the code, as each component can be tested in isolation.
However, it is important to note that VIPER is a more complex architecture than some other approaches, such as MVC or MVVM. It requires more upfront planning and can result in a larger number of classes and files in the project. As such, it may not be suitable for all projects, and it is important to carefully consider the needs and goals of the project before deciding to use VIPER.
Ultimately, the best architecture for an iOS app will depend on the specific needs and goals of the project. It is important to carefully consider all of the factors that will impact the development process and choose an architecture that is appropriate for the project.