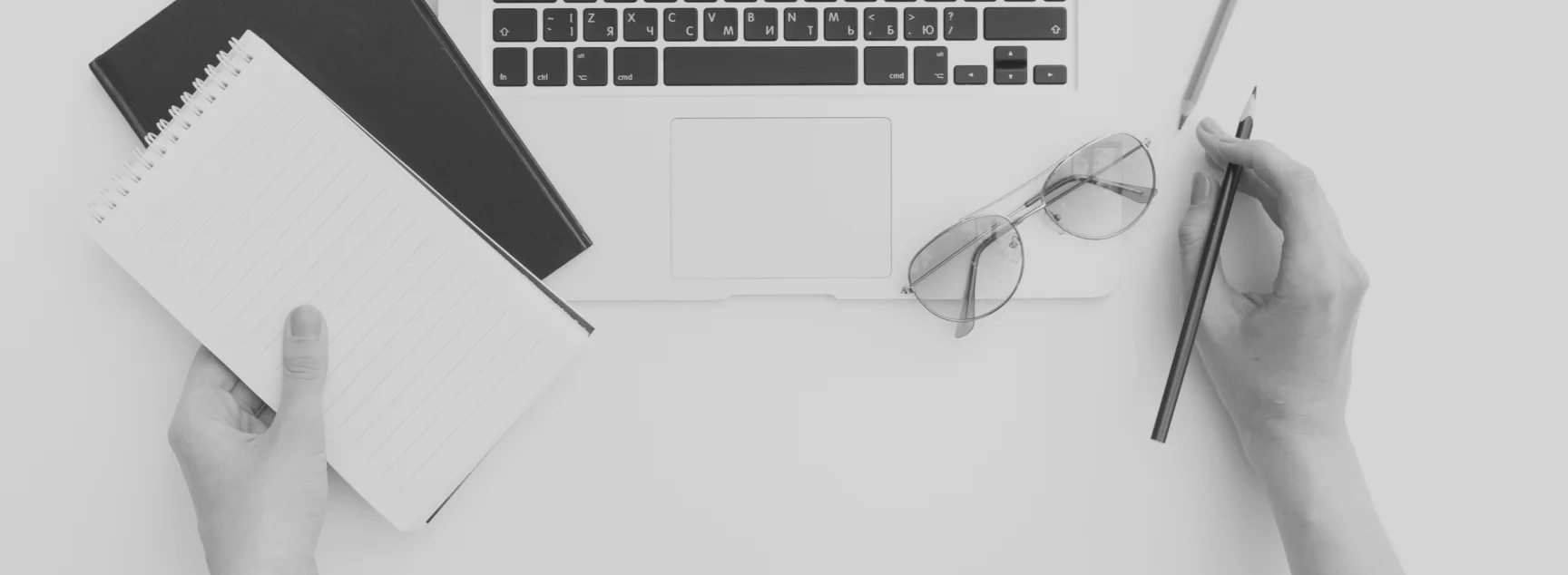
No Bad Questions About Programming
Definition of Metaprogramming
What is metaprogramming?
Metaprogramming is a programming technique that empowers programs to treat other programs as data, enabling them to dynamically manipulate or generate code during runtime or compile-time. It's like a self-updating computer program that can create or modify its own code while it's running.
Essentially, metaprogramming allows code to self-modify or create new code, offering increased flexibility, abstraction, and reusability.
What is an example of metaprogramming?
Metaprogramming can be found across various programming languages, each offering unique features to enable dynamic code manipulation. Here are some examples:
Python
- Metaclasses — Python's metaclasses allow you to customize the behavior of class creation. You can use them to enforce constraints or define custom data types dynamically, shaping how classes behave.
- Decorators — Decorators provide a concise and elegant way to modify the behavior of functions or methods. They can be used for tasks like caching, timing, or logging.
Ruby
- Open classes — Ruby lets you modify existing classes at runtime, even those from the standard library. This allows for extending built-in functionalities or building domain-specific languages (DSLs).
- Method missing — Ruby's method_missing can handle undefined method calls, enabling dynamic attributes or custom DSL implementations.
JavaScript
- eval() function — The eval() function can be used to execute JavaScript code dynamically, allowing you to create or modify code at runtime.
- Reflect object — The Reflect object provides a way to access and modify the behavior of objects, allowing for metaprogramming tasks like dynamic property access and modification.
What is the difference between metaprogramming and code generation?
In short, metaprogramming works "inside" the runtime process, whereas code generation operates "outside," generating code beforehand:
- Metaprogramming occurs during the program's runtime or compile time. It involves the program dynamically altering its behavior, and structure, or generating new code during execution.
- Code generation, on the other hand, refers to creating code at build-time or ahead of time. A separate tool or program generates source code, which is then compiled or interpreted later. While metaprogramming manipulates code dynamically, code generation produces static code that doesn't change after generation.
Key Takeaways
- Metaprogramming is a technique where programs can manipulate or generate other programs or themselves, enabling dynamic code execution or modification during runtime or compile-time.
- Examples of metaprogramming can be seen in languages like Python with metaclasses and decorators, Ruby with open classes and method_missing, and JavaScript with the eval() function and Reflect object.
- The main distinction between metaprogramming and code generation is that metaprogramming happens dynamically during runtime, while code generation occurs beforehand, producing static code that does not change after generation. Both techniques offer increased flexibility and reusability in software development.